ATM Lesson 1: Check Balance, Withdraw, and Deposit
Introduction
We will be building a very basic ATM script that will let us do some basic functions such as check our bank balance as well as deposit and withdraw. We will begin by building these three functions and a basic menu first .
Components Needed
- Little Python Brain
- A tablet/phone/laptop
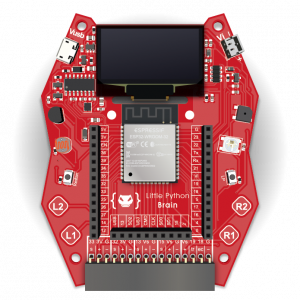
Setting the Primary Variable
First, we need to set the variable where we will store the amount of money the user has first. Let’s initiate a variable called myMoney and set it to 0.myMoney = 0
Making the checkBalance() function
Afterwards, we will define a function to return the value of myMoney. Let’s call this function checkBalance(). This a simple code where we will use the curly brackets as a placeholder for the variable we want to use. We will get into it more in later lessons when we start using more than one variable but want to make an easy to understand string.
def checkBalance(): print("Your balance is: ${} ".format(myMoney))
Making the withdraw() and deposit() functions
We will then make two more functions: one to “withdraw” money and one to “deposit” money.
Breaking it down to its most simple function: the deposit function ADDS money to our myMoney variable. On the other hand, the withdraw function SUBTRACTS money from our myMoney variable.
We can do these by taking the user input for the amount we want to deposit or withdraw, modifying the myMoney variable with an increment (+=) or decrement (-=) operator, and converting the amount the user has input to integer before we do the myMoney operation.
Afterwards, we can add feedback and provide the user with their current balance via a print function.
def deposit(): global myMoney depositAmount = input("How much would you like to deposit ($)? ") myMoney += int(depositAmount) print("Your new balance is ${}.".format(myMoney)) def withdraw(): global myMoney withdrawAmount = input("How much would you like to withdraw ($)? ") myMoney -= int(withdrawAmount) print("Your new balance is ${}.".format(myMoney))
Making a basic menu
We will then make a basic menu utilizing basic if-else conditions to check for a user input. We want to call the deposit function when the user inputs “d”, we want it to call the withdraw function when the user inputs “w”, and we want to check the balance in our account when the user inputs “c”. Any other input will simply call the menu function again. To get the user selection, we simply make a new variable called “selection” then add the string that shows the user their choices (d/w/c). the “\n” is simply a way to go a to a new line and the “>>>” is just to show the user that their input is being accepted. Afterwards, it’s just a matter of using if and elif conditions to check what the user has input.def menu(): print("Deposit - d") print("Withdraw - w") print("Check Balance - c") selection = input("d/w/c?\n >>> ") if selection is "d": deposit() elif selection is "w": withdraw() elif selection is "c": checkBalance() else: menu()
Running the code
To run the whole code, simply type menu() in a new line. This will initialize the menu function which in turn initializes the different functions we created.
menu()
The Complete Code
myMoney = 0 def checkBalance(): print("Your balance is: $ {} ".format(myMoney)) def deposit(): global myMoney depositAmount = input("How much would you like to deposit ($)? ") myMoney += int(depositAmount) print("Your new balance is ${}.".format(myMoney)) def withdraw(): global myMoney withdrawAmount = input("How much would you like to withdraw ($)? ") myMoney -= int(withdrawAmount) print("Your new balance is ${}.".format(myMoney)) def menu(): print("Deposit - d") print("Withdraw - w") print("Check Balance - c") selection = input("d/w/c?\n >>> ") if selection is "d": deposit() elif selection is "w": withdraw() elif selection is "c": checkBalance() else: menu() menu()
On the next lesson, we will put this code into a simple loop so we can do multiple “transactions”.