ATM Lesson 3: Basic Error Detection
Introduction
Now that we have a loop using just functions, we can then go a little further with our code and detect errors. For now, the most important error we have to check for is the type of data that the users will input during the deposit and withdraw functions. For now, try inputting a letter to this function and run it. Observe the error that will happen.
Components Needed
- Little Python Brain
- A tablet/phone/laptop
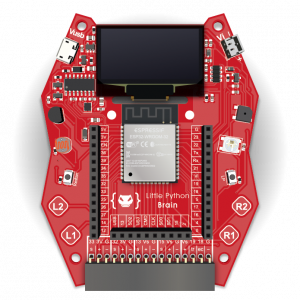
Checking for value errors in deposit and withdraw
Observe the following
Deposit - d Withdraw - w Check Balance - c d/w/c? >>> d How much would you like to deposit ($)? one hundred Traceback (most recent call last): File "/user/bank.py", line 44, in menu() File "/user/bank.p", line 33, in menu deposit() File "/user/bank.p", line 9, in deposit myMoney += int(depositAmount) ValueError: invalid literal for int() with base 10: 'one hundred' >>>
Observe the error code ValueError: invalid literal for int() with base 10: 'one hundred'
. This is is due to the inputting of a non-integer value for the deposit prompt. In order to account for this kind of user error, we can add a basic try/except condition to check for this specific error. We can edit the withdraw and deposit functions as follows:
def deposit(): global myMoney depositAmount = input("How much would you like to deposit ($)? ") try: myMoney += int(depositAmount) print("Your new balance is ${}.".format(myMoney)) except ValueError: print("Invalid value! Try again.") deposit() def withdraw(): global myMoney withdrawAmount = input("How much would you like to withdraw ($)? ") try: myMoney -= int(withdrawAmount) print("Your new balance is ${}.".format(myMoney)) except ValueError: print("Invalid value! Try again.") withdraw()
Checking for negative values in the deposit() function
Afterwards, we need to find a way to detect if a user inputs a negative value when depositing.def deposit(): global myMoney depositAmount = input("How much would you like to deposit ($)? ") try: if int(depositAmount) > 0: myMoney += int(depositAmount) print("Your new balance is ${}.".format(myMoney)) else: print("Please enter a value greater than 0!") except ValueError: print("Invalid value! Try again.") deposit()
Checking for insufficient balance upon using the withdraw() function
Similar to the previous We will then add another algorithm to check for the balance BEFORE processing the withdraw function. We can edit the withdraw() function as follows:def withdraw(): global myMoney withdrawAmount = input("How much would you like to withdraw ($)? ") try: if int(withdrawAmount) < myMoney: myMoney -= int(withdrawAmount) print("Your new balance is ${}.".format(myMoney)) else: print("Insufficient balance!") withdraw() except ValueError: print("Invalid value! Try again.") withdraw()
The Complete Code
myMoney = 0 def checkBalance(): print("Your balance is: $ {} ".format(myMoney)) def deposit(): global myMoney depositAmount = input("How much would you like to deposit ($)? ") try: if int(depositAmount) > 0: myMoney += int(depositAmount) print("Your new balance is ${}.".format(myMoney)) else: print("Please enter a value greater than 0!") except ValueError: print("Invalid value! Try again.") deposit() def withdraw(): global myMoney withdrawAmount = input("How much would you like to withdraw ($)? ") try: if int(withdrawAmount) < myMoney: myMoney -= int(withdrawAmount) print("Your new balance is ${}.".format(myMoney)) else: print("Insufficient balance!") withdraw() except ValueError: print("Invalid value! Try again.") withdraw() def prompt(): prompt = input("Would you like to make another transaction? (y/n) \n>>>") if prompt is "y": menu() elif prompt is "n": pass else: prompt() def menu(): print("Deposit - d") print("Withdraw - w") print("Check Balance - c") selection = input("d/w/c?\n>>> ") if selection is "d": deposit() elif selection is "w": withdraw() elif selection is "c": checkBalance() else: menu() prompt() #Initializes the prompt() function menu()
On the next lesson, we will put this code into a simple loop so we can do multiple “transactions”.